티스토리 뷰
1. 프로젝트 생성
비쥬얼스튜디오 코드 실행
새 터미널 창을 열어 폴더를 생성합니다. 여기서는 프로젝트 폴더명을 movie 폴더로 하겠습니다.
mkdir 프로젝트폴더명
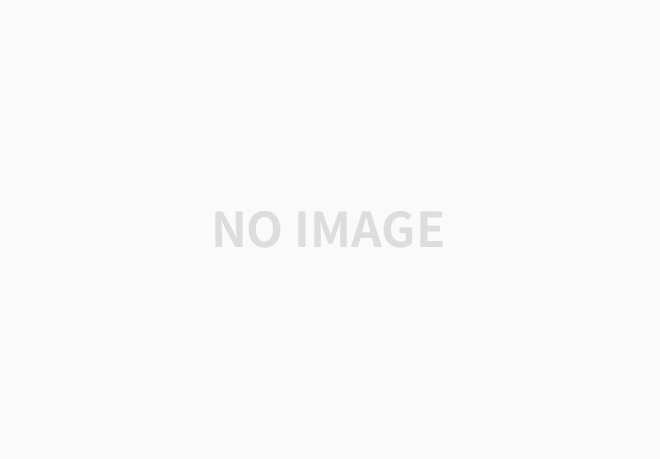
생성한 폴더로 경로 이동을 하여, 개발 환경 세팅을 합니다.
cd movie
npm install -g yarn
npm install -g @vue/cli
프로젝트 생성
vue create frontend // frontend 폴더 생성
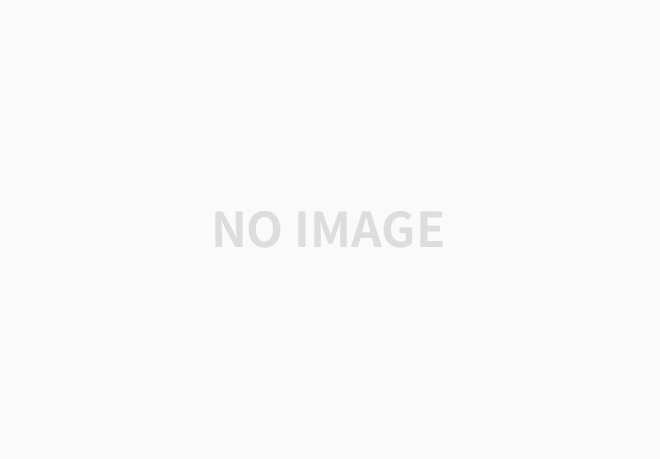
서버실행
cd frontend
npm run serve //서버 실행
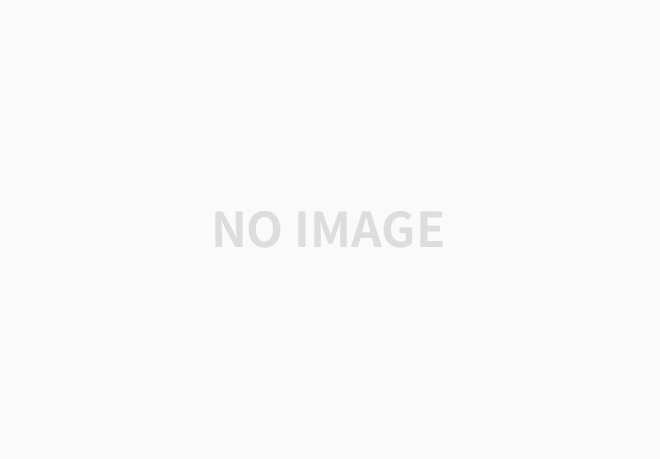
익스플로러를 열어 locahost:8080으로 접속하면 아래와 같은 화면을 확인할 수 있습니다.
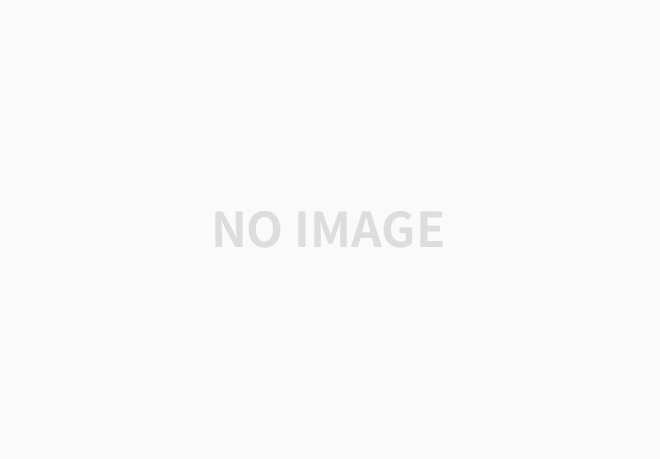
2. 백엔드 개발환경 세팅
최상위폴더 movie로 이동한 후 express 프레임워크를 설치
cd ../
npm install -g express-generator
express --view=pug backend // backend 폴더 생성
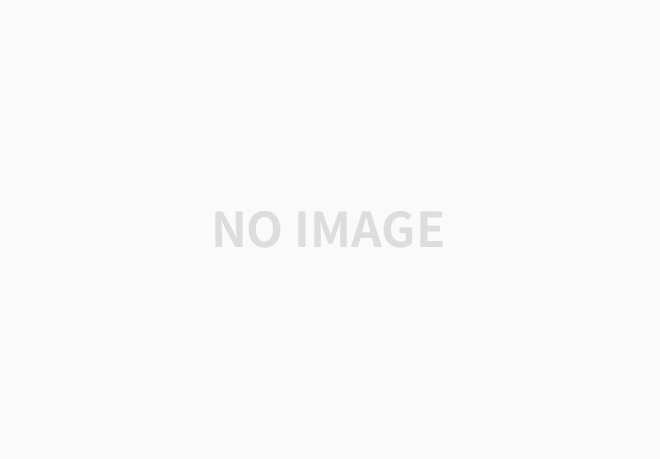
서버 실행
cd backend
npm install
SET DEBUG=backend:*
npm start // 서버 실행
익스플로러를 열어 localhost:3000 으로 접속하면 아래와 같은 화면을 확인할 수 있습니다.
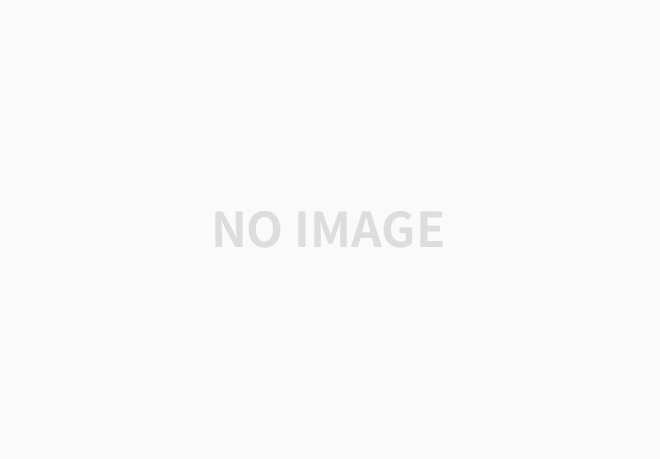
Ctrl+C를 눌러 서버를 중지합니다. Y
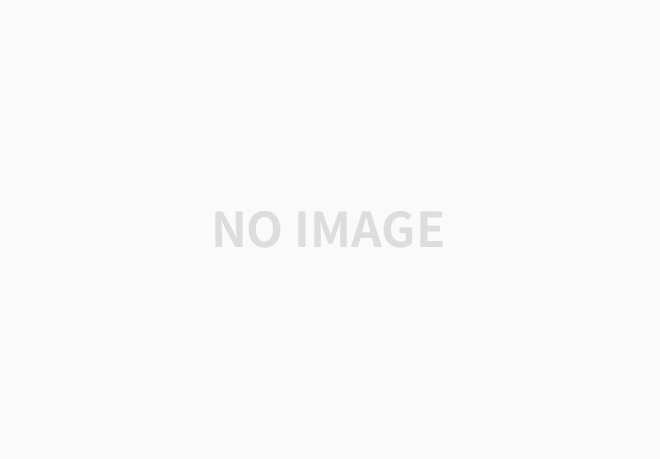
3. 백엔드와 연동
Vue-cli 3이상 버전에서는 project 생성 시 webpack 설정 파일이 내부로 감춰져 있습니다.
node_modules\@vue\cli-service\webpack.config.js
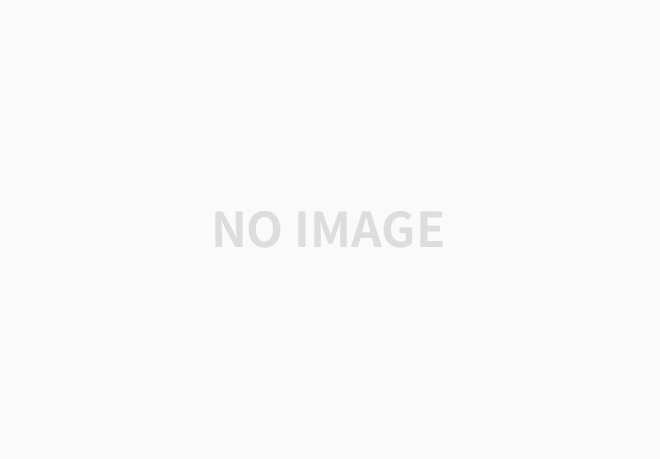
아래 설정을 하려면 해당 파일을 프로젝트 폴더에 생성하면 됩니다.
frontend 폴더로 이동하여, vue.config.js를 생성한 뒤 아래와 같이 입력합니다.
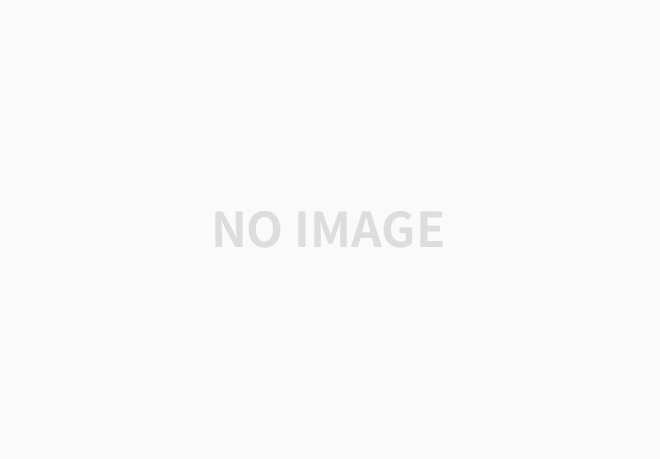
module.exports = {
devServer: { //① api 요청이 있을때 어디에서 처리할지를 설정
proxy: {
'/api': {
target: 'http://localhost:3000/api',
changeOrigin: true,
pathRewrite: {
'^/api': ''
}
}
}
},
outputDir: '../backend/public', //② 배포 파일의 위치를 지정
}
참조
cli.vuejs.org/config/#global-cli-config
Configuration Reference | Vue CLI
Configuration Reference Global CLI Config Some global configurations for @vue/cli, such as your preferred package manager and your locally saved presets, are stored in a JSON file named .vuerc in your home directory. You can edit this file directly with yo
cli.vuejs.org
frontend 폴더에서 npm run build를 실행합니다.
cd frontend
npm run build
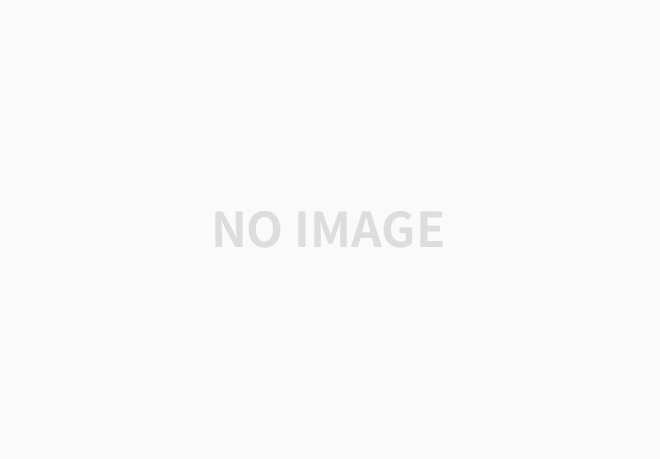
위 빌드 명렁어를 입력하면 backend/public 폴더의 구조가 변경된 것을 확인할 수 있습니다.
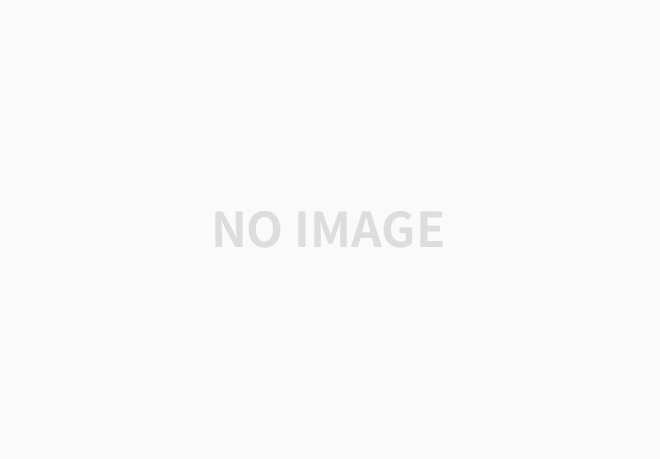
그리고 나서 Express 서버를 실행시키면, localhost:3000의 페이지도 프론트엔드쪽과 동일하게 변경된 것을 확인할 수 있습니다.
cd ../backend
npm start
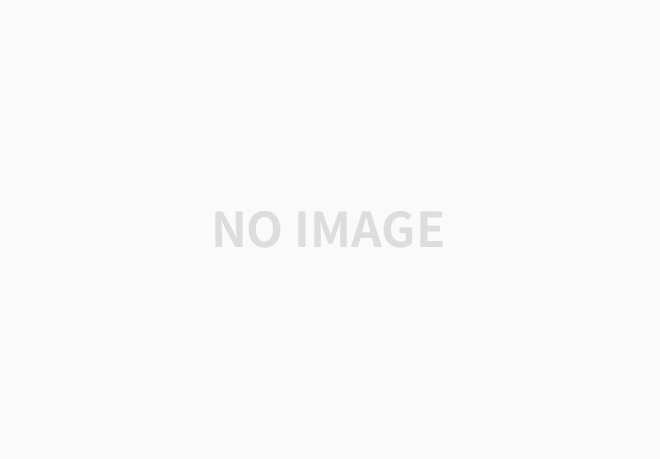
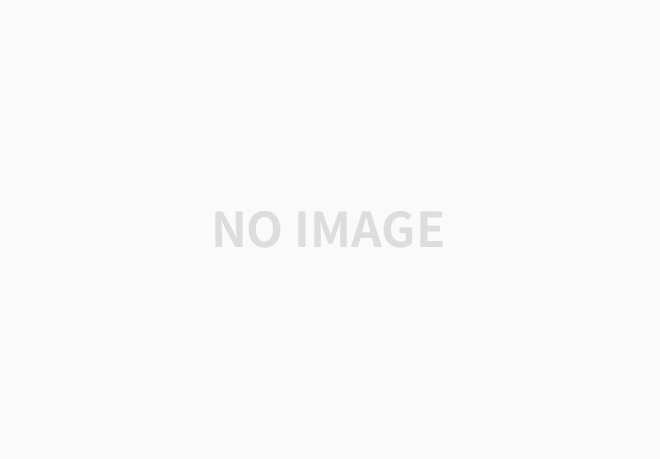
4. 백엔드에서 프론트엔드로 보낼 데이터 마련하기
backend 폴더 하위에 movies.json파일을 아래와 같이 생성합니다.
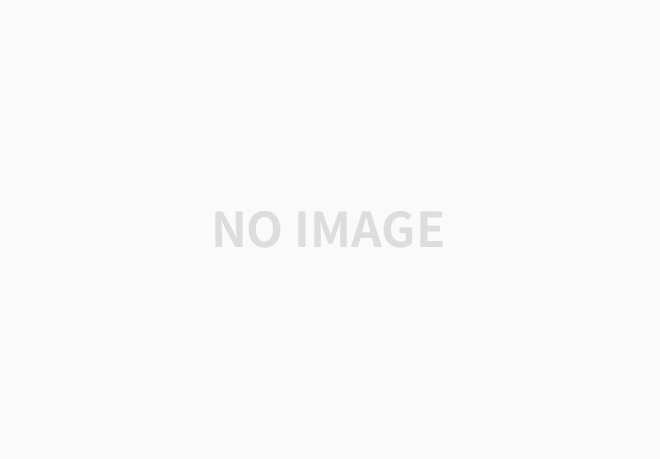
movies.json에 네이버 영화 데이터를 입력합니다.
이제 라우터를 설정합니다. backend/router/ 하위에 movies.js 를 생성한 뒤 다음과 같이 입력합니다.
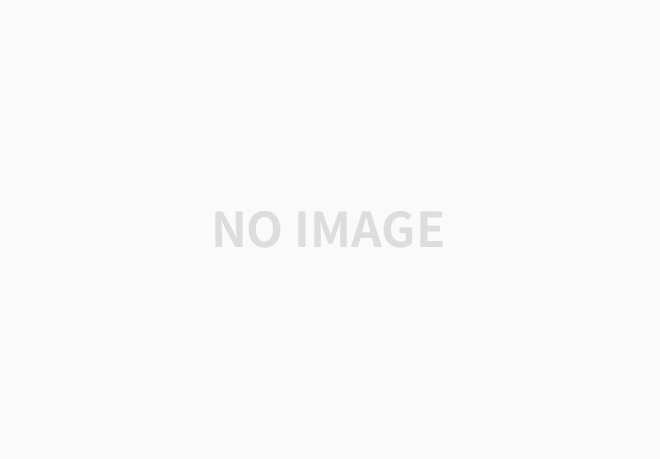
var express = require('express');
var router = express.Router();
var movies = require('../movies.json');
router.get('/', function (req, res, next) {
res.send(movies)
});
// 영화 상세 페이지를 위한 코드
router.get('/:id', function (req, res, next) {
var id = parseInt(req.params.id, 10)
var movie = movies.filter(function (movie) {
return movie.id === id
});
res.send(movie)
});
module.exports = router;
backend/app.js에는 아래의 내용을 추가합니다.
var moviesRouter = require('./routes/movies');
...
app.use('/api/movies', moviesRouter);
프론트엔드쪽에서 /api/movies 요청을 보내면, 위 코드에서 설정한 라우터를 통해 데이터를 처리합니다.
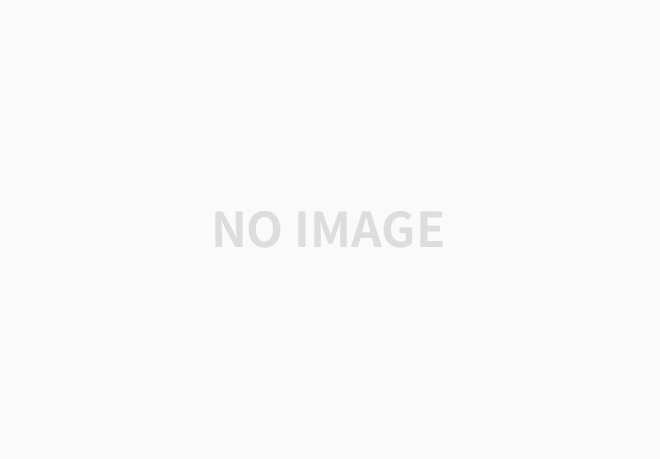
5. 프론트 데이터 바인딩 화면 제작
frontend/src/components 하위에 .vue 뷰 파일을 생성하고 (여기서는 임의 파일명 MovieIndexPage.vue)
다음과 같이 입력합니다.
<template>
<div class="app">
<h1>5월 마지막주 영화 예매 순위</h1>
<ul class="movies">
<li v-for="movie in movies" :key="movie.id" class="item">
<span class="rank">{{ movie.id }}</span>
<router-link :to="{ name: 'show', params: { id: movie.id } }">
<img v-bind:src="movie.poster" class="poster" />
</router-link>
<div class="detail">
<strong class="tit">{{ movie.name }}</strong>
<span class="rate"
>예매율 <span class="num">{{ movie.rate }}</span></span
>
<router-link
:to="{ name: 'show', params: { id: movie.id } }"
class="link"
>자세히보기</router-link
>
</div>
</li>
</ul>
</div>
</template>
<script>
export default {
created () {
// 컴포넌트가 생성될 때, /api/movies에 요청을 보냅니다.
this.$http.get('/api/movies')
.then((response) => {
this.movies = response.data
}).catch(err => {
alert(err);
console.log(err);
})
},
data () {
return {
movies: []
}
}
}
</script>
마찬가지로 frontend/src/components 하위에
상세보기 뷰 페이지를 생성하고 아래와 같이 입력합니다. (여기서는 임의 파일이름 MovieDetailPage.vue)
<template>
<div class="detail">
<h1>{{movie.name}}</h1>
<img v-bind:src="movie.poster" class="poster">
<section>
<h2>영화정보</h2>
<dl class="info">
<dt>감독</dt>
<dd>{{movie.director}}</dd>
<dt>출연</dt>
<dd>{{movie.actors}}</dd>
<dt>러닝타임</dt>
<dd>{{movie.time}}</dd>
</dl>
</section>
<section>
<h2>줄거리</h2>
<p v-html="movie.synopsis" class="synopsis"></p>
</section>
<router-link :to="{ name: 'index', params: { id: movie.id }}" class="link">돌아가기</router-link>
</div>
</template>
<script>
export default {
created: function () {
var id = this.$route.params.id;
this.$http.get('/api/movies/${id}')
.then((response) => {
this.movie = response.data[0]
})
},
data: function () {
return {
movie: {}
}
}
}
</script>
이제 라우터를 설정합니다.
frontend/src/routers/index.js 열어 아래와 같이 입력합니다.
import Vue from 'vue'
import VueRouter from 'vue-router'
import Index from '@/components/MovieIndexPage'
import Show from '@/components/MovieDetailPage'
Vue.use(VueRouter)
const routes = [
{
path: '/',
name: 'index',
component: Index
},
{
path: '/:id',
name: 'show',
component: Show
}
]
const router = new VueRouter({
routes
})
export default router
라우터를 설정했으므로, frontend/src/app.vue를 열어 아래와 같이 수정합니다.
<template>
<div id="app">
<router-view/>
</div>
</template>
API 통신을 위해 ROOT 경로로 가서 npm install axios --save 명령을 실행하여, axios 패키지를 설치합니다.
npm install axios --save
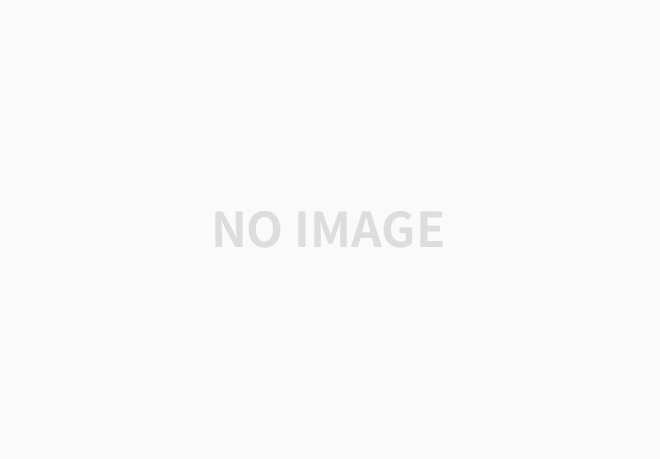
설치 완료 후 frontend/src/main.js를 다음을 추가합니다.
..
import axios from 'axios'
..
Vue.prototype.$http = axios;
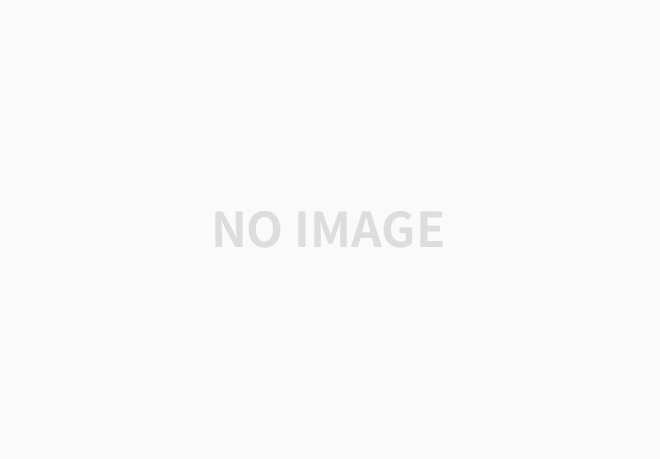
빌드 후 실행해 봅니다.
cd ..\frontend\
npm run build
cd ..\backend\
npm start
http://localhost:3000/ 브라우저를 열어 실행해보면 아래와 같이 실행되면 연동이 완료된 것을 확인 할 수 있습니다.
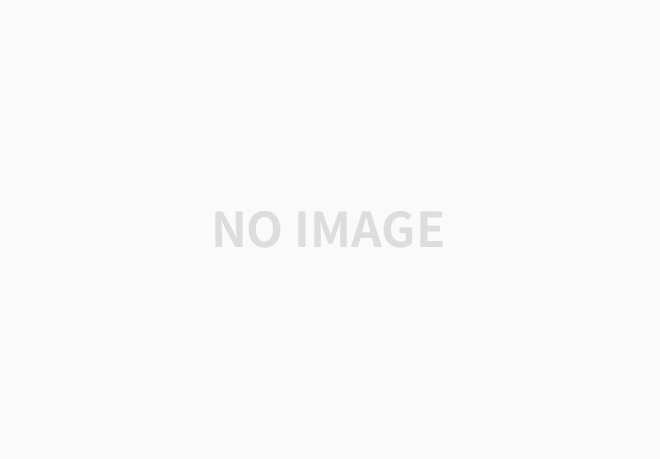
이 과정으로 프론트엔드와 백엔드가 어떻게 데이터를 주고받는지 알 수 있었습니다.
다음글에서 본격적으로 정적인 데이터가 아닌 Express + MySQL로 DB 연동을 통해 동적 데이터 다루는 법을 소개하겠습니다.
다음글
marshmello.tistory.com/65?category=1158454
[PWA] Vue.js + Express + MySQL 연동 2
저번글에서는 vue.js와 Express로 프론트엔드와 백엔드 간의 통신 방법을 다뤄보았습니다. marshmello.tistory.com/64 [PWA] Vue.js + Express + MySQL 연동 1 1. 프로젝트 생성 비쥬얼스튜디오 코드 실행..
marshmello.tistory.com
'WEB' 카테고리의 다른 글
vis.js 네트워크 라이브러리 및 샘플코드 다운로드 (0) | 2024.09.27 |
---|---|
[PWA] Vue.js + Express + MySQL 연동 2 (12) | 2021.01.06 |
kboard default 테마 디자인 수정 (0) | 2020.12.29 |
한국전자인증 도메인 연결 방법 (0) | 2020.12.22 |
aws ec2 서버와 카페24 도메인 연결 (0) | 2020.12.22 |
- Total
- Today
- Yesterday